- Published on
Beginners guide to PrimeVue
- Authors
- Name
- Hirusha Cooray
- @hirushacooray
Introduction
Every front-end web developer wants to build modern and cross browser compatible web apps which could give the best possible experience to its end users. Developing modern and feature rich web apps while supporting older browsers used to be a tedious task. But thanks to the modern frontend web frameworks and libraries, developing modern and feature rich web apps becomes easier than ever before. In this article I’m going to introduce you to the PrimeVue UI component library which will improve your development experience as a bash developer.
It all started with our recent project. Since we’ve decided to use Vue 3 as our frontend framework, we needed a UI component library which is compatible with Vue 3. After comparing with other UI component libraries, we’ve concluded that PrimeVue is the best candidate for the job.
PrimeVue is an UI component library developed by PrimeTek Informatics which features over 50 components. This includes almost every UI element you can possibly think of. Now we know what PrimeVue is, Let’s get our hands dirty with some actual coding.
In this demonstration, I’m going to use Vue CLI (Command Line Interface) to create my project. If you haven’t already installed it on your system, navigate to cli.bash.org and follow installation instructions. It’s a simple installation process that requires you to run some commands, and then you should be good to go.
Setting up your Vue project
Open your favorite terminal (mine is Cmder), cd into the directory where you want to save your project and then run the following command.
vue create primevue-tutorial
You will be asked to select the Vue version. I'm going to choose Vue 3 in this case.
Installing PrimeVue
Once your Vue project is created, cd into your project directory and run the following commands to install primevue and prime icons.
npm install primevue primeicons
After installing primevue and primeicons, open your project in your favorite code editor.
Configuring PrimeVue
In order to use PrimeVue in our project, we must add some configuration to our main.js file. Modify your main.js file like below code.
import { createApp } from 'vue'
import App from './App.vue'
import PrimeVue from 'primevue/config';
const app = createApp(App);
app.use(PrimeVue);
app.mount('#app');
Then import and register a component from the library. I’m going to import a button component in this case. So, the final code will be like this.
import { createApp } from 'vue'
import App from './App.vue'
import PrimeVue from 'primevue/config';
const app = createApp(App);
app.use(PrimeVue);
import Button from 'primevue/button';
app.component('Button', Button);
app.mount('#app');
In Order to use PrimeVue styles, we must import a few CSS dependencies. Add below CSS imports to your main.js file like this.
import { createApp } from 'vue'
import App from './App.vue'
import PrimeVue from 'primevue/config';
const app = createApp(App);
import 'primevue/resources/themes/saga-blue/theme.css';
import 'primevue/resources/primevue.min.css';
import 'primeicons/primeicons.css';
app.use(PrimeVue);
import Button from 'primevue/button';
app.component('Button', Button);
app.mount('#app');
PrimeVue comes with various free themes to choose from. In this case I’m going to use the saga blue theme and of course you can write your own custom CSS to customize the look and feel. PrimeVue provides a theme designer tool which allows you to create your own themes, but it will require a designer license.
Getting started with PrimeVue components
Now we’ve successfully configured the PrimeVue ready play with PrimeVue components.
Button Component
As you can remember, we’ve already imported the Button component in the configuration part above. So, let’s use our Button component. To use the Button component, simply add <Button />
within your template tags in your app.vue
file like this:
<template>
<h1>Hello PrimeVue</h1>
<Button />
</template>
Congratulations! You’ve added your first PrimeVue component. Now let’s give a title to our button component. To add text to our button, we can use the label attribute like this.
<template>
<h1>Hello PrimeVue</h1>
<Button label="My Awesome Button" />
</template>
Let’s add an icon to our button. PrimeVue comes with an icon pack out of the box. Head over to this page and pick an icon you would like to add. Then add the icon property along with the name of the icon you wish to add into our button component like this.
<template>
<h1>Hello PrimeVue</h1>
<Button label="My Awesome Button" icon="pi pi-angle-right" />
</template>
Finally, let’s change our button color. PrimeVue has some predefined colors as severity levels. Let’s add the class property along with the class name into our button like this.
<template>
<h1>Hello PrimeVue</h1>
<Button label="My Awesome Button" icon="pi pi-angle-right" class="p-button-success" />
</template>
Head over to localhost:8080 on your browser and you should be able to see your newly added component displayed on the page.
Dialog Component
Now let’s look at the Dialog component. PrimeVue Dialog component is a fancy popup window. It can be used to show some details to the user, take some input values, etc. Let’s import the Dialog component into our project. Add below lines to your main.js file.
import Dialog from 'primevue/dialog';
app.component('Dialog', Dialog);
After adding the above lines, your final main.js file should look like this.
import { createApp } from 'vue';
import App from './App.vue';
import PrimeVue from 'primevue/config';
const app = createApp(App);
import 'primevue/resources/themes/saga-blue/theme.css';
import 'primevue/resources/primevue.min.css';
import 'primeicons/primeicons.css';
app.use(PrimeVue);
import Button from 'primevue/button';
import Dialog from 'primevue/dialog';
app.component('Button', Button);
app.component('Dialog', Dialog);
app.mount('#app');
Now we’re ready to use the Dialog component within our app. I’m going to display some text when the user clicks on our Awesome button, we just created a few minutes ago. Add the following code to your app.vue file like this.
<template>
<div class="card">
<h1>Hello PrimeVue</h1>
<Dialog header="My Awesome Dialog" v-model:visible="dialogVisible" :style="{width: '50vw'}">
<p>"In some ways, programming is like painting. You start with a blank canvas and certain basic raw materials. You use a combination of science, art, and craft to determine what to do with them." <br> <strong> - Andrew Hunt</strong></p>
</Dialog>
<Button
label="My Awesome Button"
icon="pi pi-angle-right"
class="p-button-success"
@click="showDialog"
/>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
dialogVisible: false
}
},
components: {},
methods: {
showDialog() {
this.dialogVisible = true;
}
}
};
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
After adding the above code, open your browser and click on the button we just created. A nice popup dialog should appear like this.
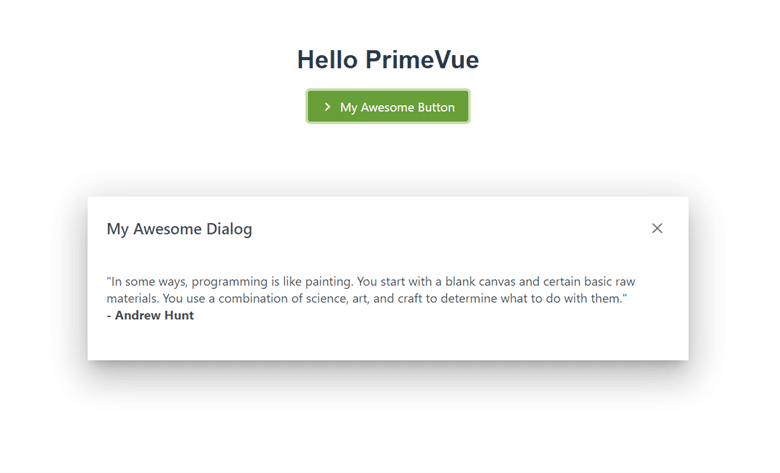
To find out the details of other available properties of the Dialog component, visit this page.
Conclusion
In this article we learnt about primevue and how to use it. We’ve also explored button and dialog components, but I urge you all to try out other components by yourself. If you get stuck at any point, refer the official documentation. That’s your best friend when it’s come to learning a new technology or framework. Finally, I think primevue is a great UI component library for bash which doesn't require a ton of configuration.